设置背景图还是挺简单的,直接container里加个decration然后加个图片就行了,就像这样
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
|
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Container(
decoration: BoxDecoration(
image: DecorationImage(
image: NetworkImage(
'https://img.zcool.cn/community/0372d195ac1cd55a8012062e3b16810.jpg'),
fit: BoxFit.cover,
),
)));
}
}
|
![/wp-content/uploads/2019/02/flutter_bg1.png]()
可以看到,appbar的部位并没有背景图,我们要做的就是全屏透明,把appbar那部分也要透明带上背景,这个需求也很简单
代码如下
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
|
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
image: DecorationImage(
image: NetworkImage(
'https://img.zcool.cn/community/0372d195ac1cd55a8012062e3b16810.jpg'),
fit: BoxFit.cover,
)),
child: Scaffold(
backgroundColor: Colors.transparent, //把scaffold的背景色改成透明
appBar: AppBar(
backgroundColor: Colors.transparent, //把appbar的背景色改成透明
// elevation: 0,//appbar的阴影
title: Text(widget.title),
),
body: Center(
child: Text('Hello World'),
)));
}
}
|
效果长这样
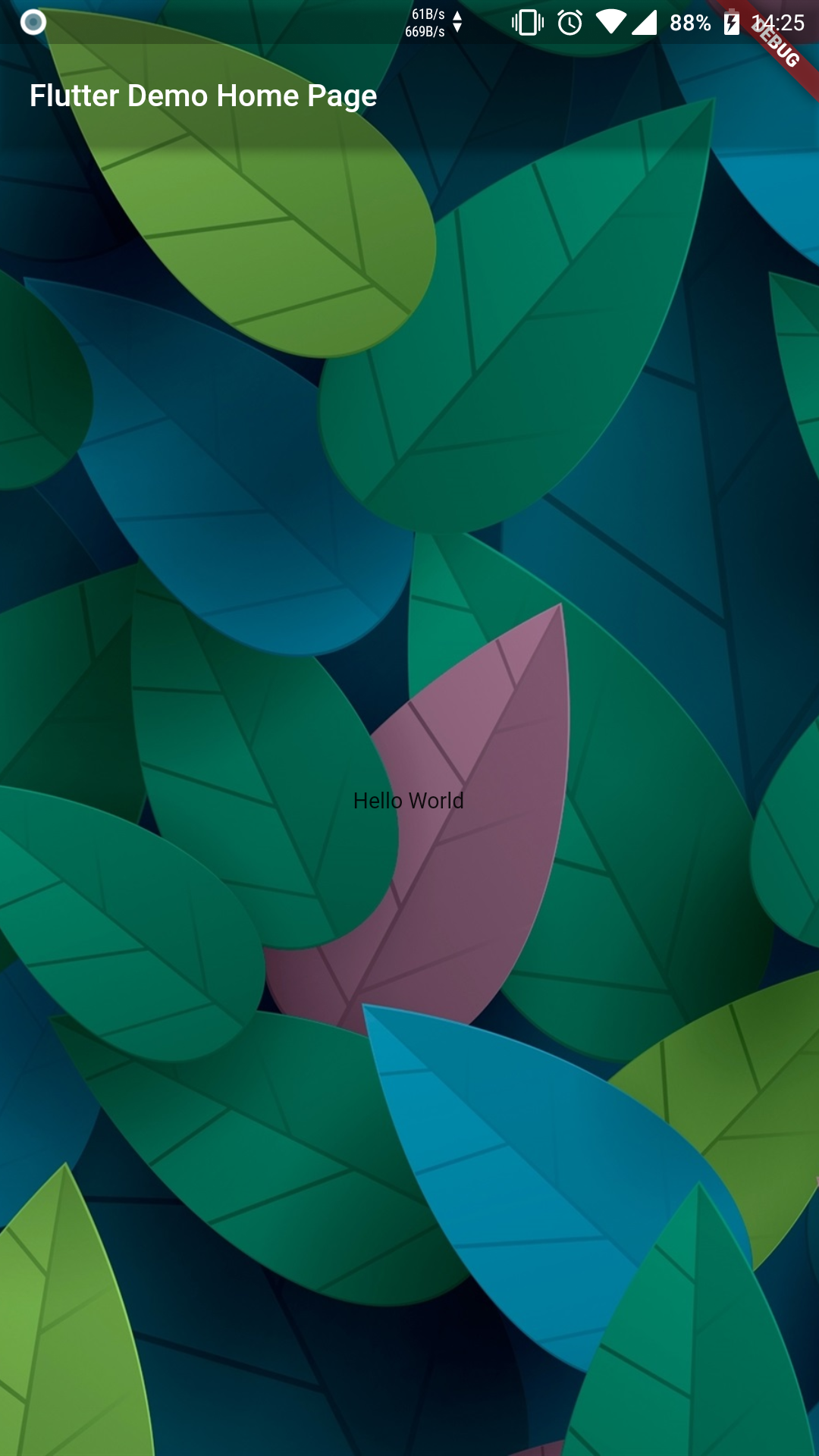
这就达到了我们想要的效果了,可以看到appbar那里还有个阴影,要去掉的话只要把上面代码的
1
|
// elevation: 0,//appbar的阴影
|
注释掉就行了
那么状态栏的全透明沉浸呢
在 main.dart 的 runapp 后面加上
1
2
|
SystemUiOverlayStyle systemUiOverlayStyle = SystemUiOverlayStyle(statusBarColor: Colors.transparent);
SystemChrome.setSystemUIOverlayStyle(systemUiOverlayStyle);
|
就可以了
状态栏沉浸来自
https://www.jianshu.com/p/97e93c82ccef